Model
Introduction
A model in D3E Studio is a fundamental component that defines the structure and characteristics of data within your application. It acts as a blueprint for creating, storing, and interacting with information.
Representing objects containing application data, models play a crucial role in shaping both the backend functionality and user interface.
This comprehensive description outlines the key aspects and functionalities associated with models.
Example: Ticket, Agent, User
Key Components of Model
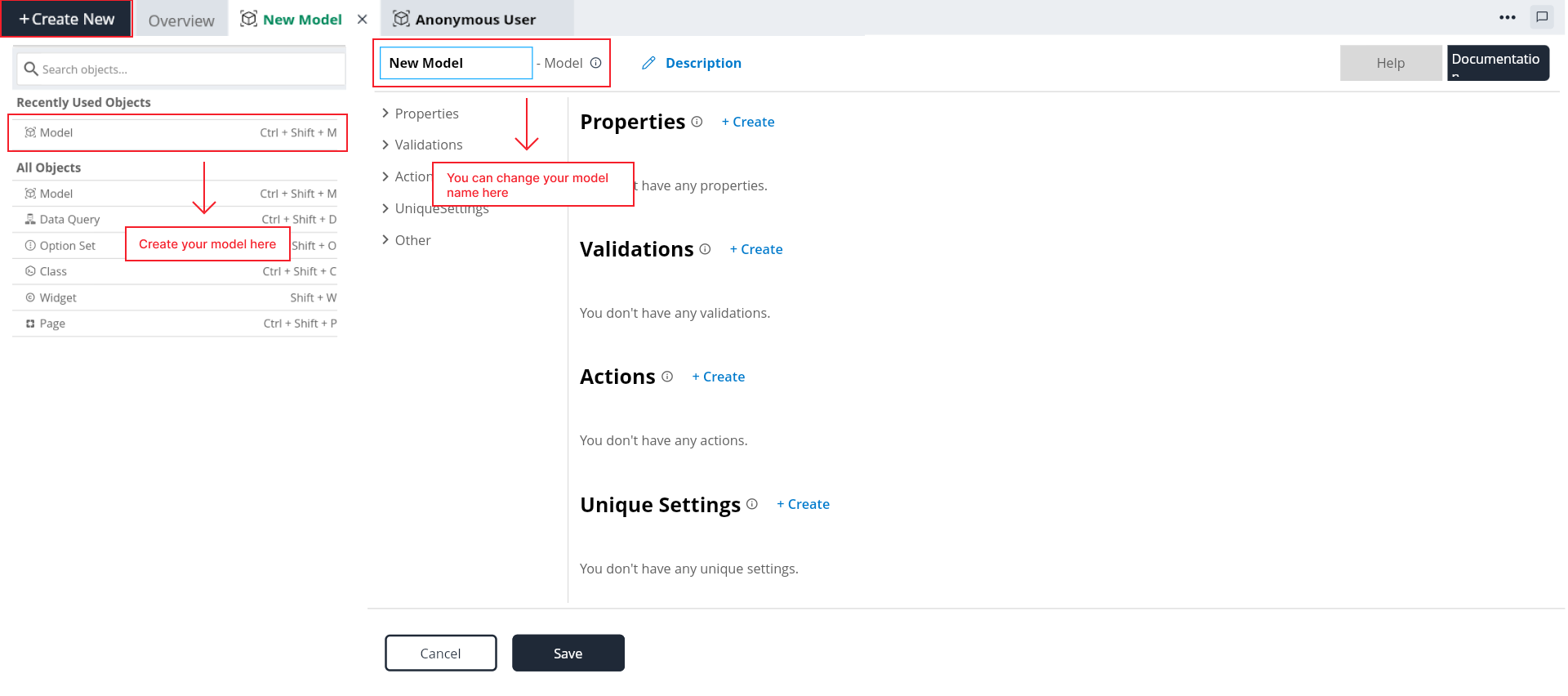
Name:
- Every model is uniquely identified by a name within the API.
Description
- Here you can give a detailed and informative explanation or representation of Model. It is a narrative that provides additional information to help understand, identify, or explain about the model.
Documentation
- Allows users to document and store information about specific Model in the project. Write notes, explanations, or guidelines related to Model. Facilitates collaboration by providing a shared space for notes and documentation.
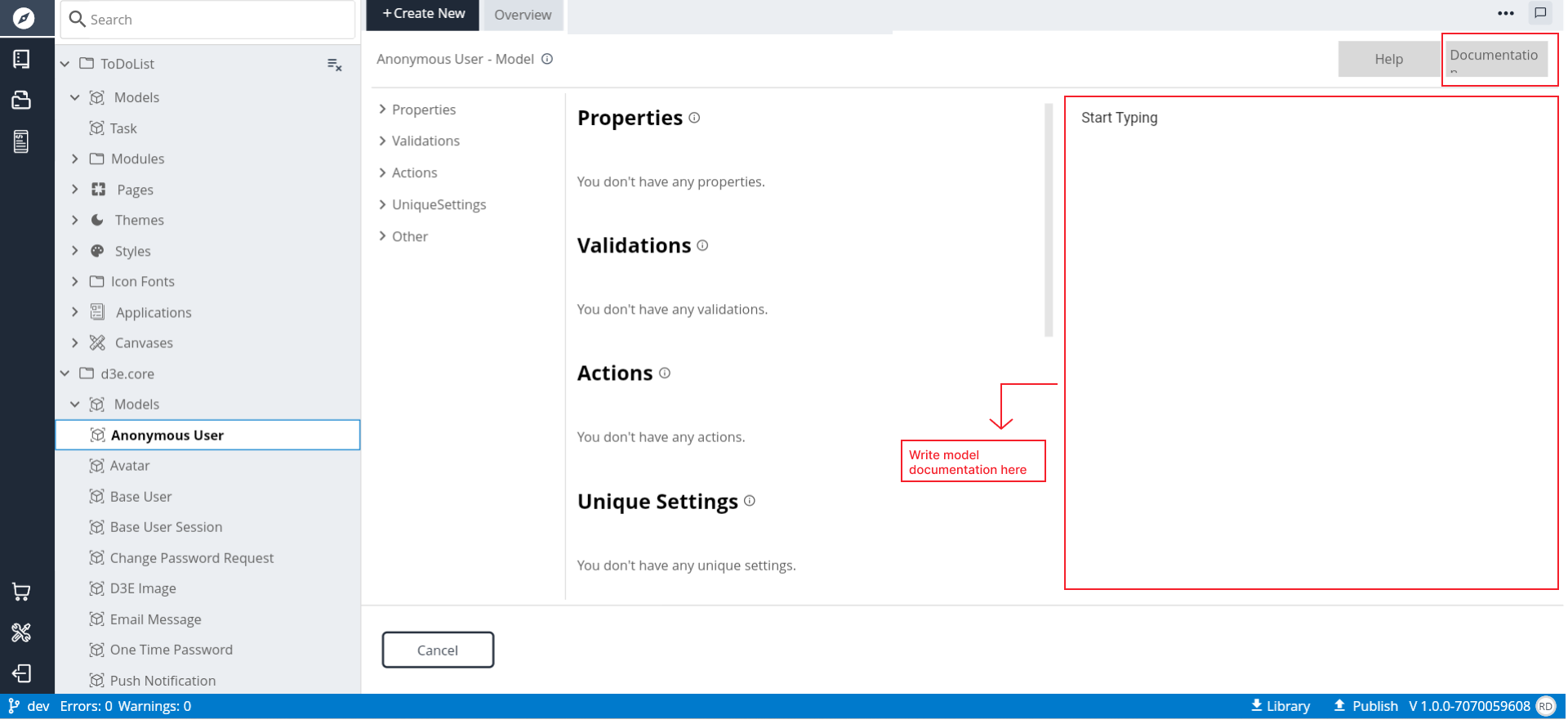
Properties:
- Describes the attributes or fields of the data object.
- Specifies the various characteristics and data types associated with the model.
- Each property encapsulates a specific piece of data within the object, providing a structured way to organize and describe the information.
Key Components of Properties:
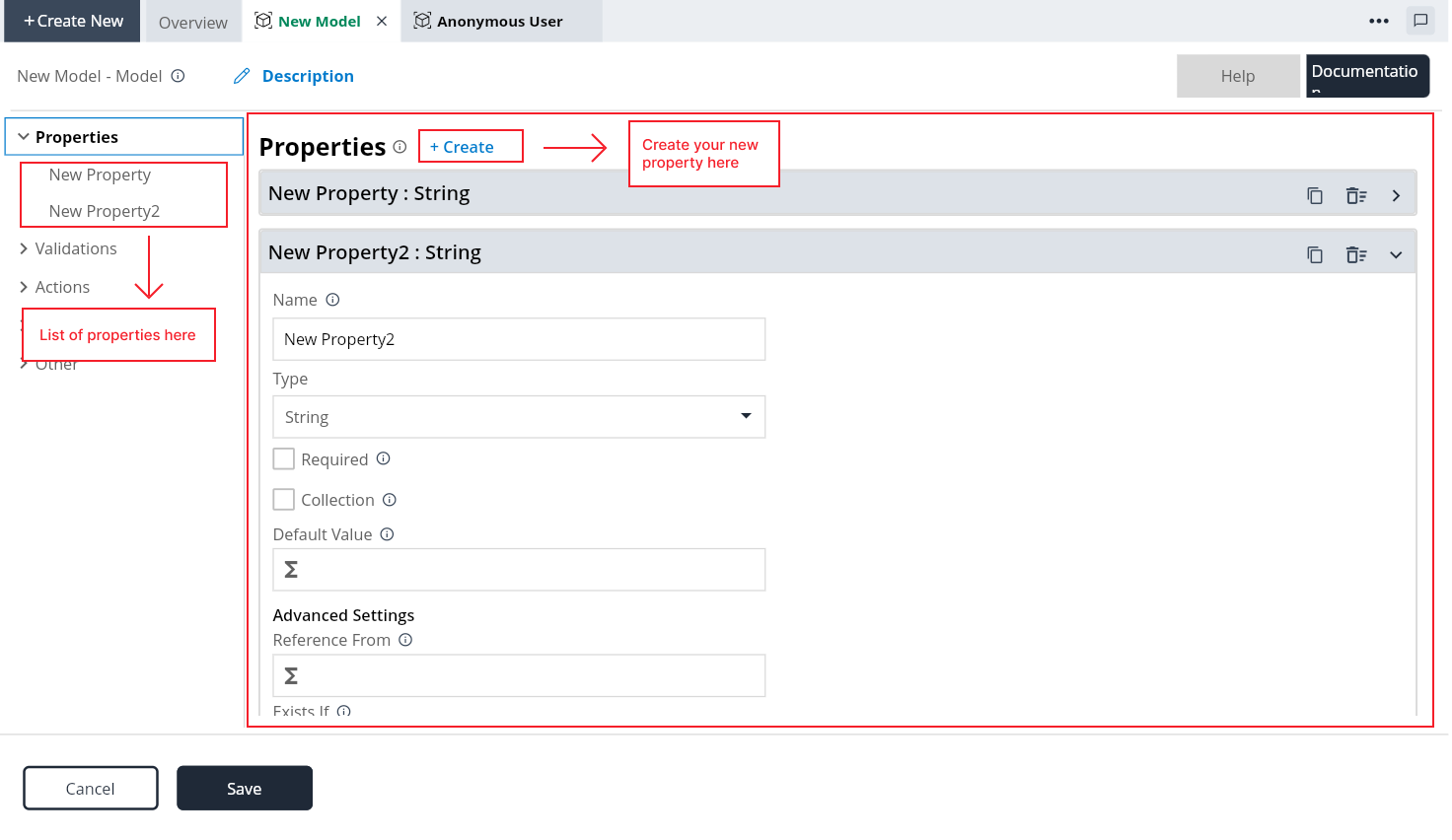
Name:
- The name of the property serves as its identity within the model. It uniquely identifies the specific data field.
Type
The type of a property specifies the kind of data it can hold. It can be a primitive data type, another model, or an option set.
Significance: Type define the nature of the data the property can store, ensuring consistency and enabling D3E Studio to generate appropriate backend structures.
- name: "firstName" type: "String" - name: "age" type: "Integer" - name: "Address" type: "AddressModel" # Another model as a type - name: "Gender" type: "GenderOptionSet" # Option set as a type
Required
The "required" property in models specifies whether a particular property must have a value assigned. If a property is marked as required, it means that when creating or updating an object based on this model, a value for this property must be provided, and leaving it empty would result in a failure, ensuring essential data is captured.
Significance: Marking a property as required ensures that crucial data is captured, enhancing the reliability and completeness of the information stored in the object
properties: - name: "email" type: "String" required: true - name: "password" type: "String" required: true - name: "phoneNumber" type: "String" required: false
This property is a list(multiple Entries)
This property in models indicates that a particular property can hold multiple values, essentially turning it into a list or an array. This is useful when a property needs to represent a collection of items rather than a single value.
Significance: Marking a property as a collection allows it to store multiple instances of the specified type, accommodating scenarios where multiple items are associated with a single entity.
properties: - name: "subjects" type: "Subject" collection: true - name: "Mobile Number" type: "Integer" collection: true - name: "Awards" type: "String" collection: false
Default Value
The "default value" property in D3E Studio models allows you to specify a predefined value that a property should take if no explicit value is provided during object creation. This default value serves as a fallback, ensuring that the property always has a valid initial value even if not explicitly set.
Significance: Default values provide a way to initialize properties, ensuring they have meaningful initial data even when not explicitly set.
properties: - name: "status" type: "String" default: "Active" - name: "priority" type: "Integer" default: 1 - name: "category" type: "String"
Type Default Value String null Boolean false Integer 0 Double 0.0 Date null Time null File null Duration null
Reference From
The concept of referenceFrom refers to specifying that a property in a model is referencing another property within the same model. This is useful when you want to establish relationships or associations between different properties within the same model.
Independence: Reference properties are independent entities. They can exist on their own and typically represent a connection to another entity without directly embedding the referenced data.
Use Case: Reference properties are useful when you want to establish relationships between different models without duplicating the data.
properties: - name: "parentTask" type: "TaskModel" referenceFrom: "tasks" # Indicates that this property references the "tasks" property in the same model - name: "tasks" type: "String"
Exists If
The "Exists-if" attribute in model properties provides a conditional mechanism that determines whether a property should hold a value based on a specified condition. If the condition evaluates to true, the property holds the specified value; otherwise, it defaults to a predefined default value.
Significance: This attribute is useful for making a property's value conditional on certain criteria, providing flexibility in determining when a property should be populated.
properties: - name: "discountPercentage" type: "Integer" exists-if: "customerType == 'Premium'" default: 0 - name: "customerType" type: "String"
Description
- Here you can give a detailed and informative explanation or representation of Property. It is a narrative that provides additional information to help understand, identify, or explain about the property.
Read Type
"Read Type" in D3E Studio is a feature related to access control, specifically governing permissions for reading or writing objects within a model.
Types of Read Type:
- Read and Write
- Write Once
- Read Only
- Local
Overriding
Computed
The term "Computed" typically refers to computed properties or computed fields within a model. Computed properties allow you to define dynamic values that are calculated based on the values of other properties within the same model. These computed properties are not stored directly in the database but are calculated on-the-fly when requested.
Significance: Computed properties are useful for deriving values dynamically, performing calculations, or aggregating data without directly storing the computed result in the database.
Model: name: "Invoice" properties: - name: "quantity" type: "Integer" - name: "price" type: "Double" - name: "total" type: "Double" computed: "quantity * price"
Transient
The term "Transient" is often used in the context of defining properties in models. A transient property is a property that is not persisted or stored in the database. It exists temporarily during the execution of certain actions or processes but is not permanently saved to the data storage.
Significance: Transient properties are useful for representing temporary or calculated values that do not need to be stored permanently but are needed for a specific operation or calculation.
Model: name: "Employee" properties: - name: "firstName" type: "String" - name: "lastName" type: "String" - name: "fullName" type: "String" transient: true computed: "firstName + ' ' + lastName"
Child
A child property is a property that is conceptually a part of another entity, often referred to as the parent entity. Child properties are dependent on the existence of the parent entity.
Independence: Child properties do not exist independently. They are part of the parent entity and are typically saved or deleted along with the parent.
Use Case: Child properties are useful when you have a hierarchical or composition relationship, and the child properties are closely tied to the existence of the parent entity.
Unique
- The unique property in a model is used to define uniqueness constraints on a specific property. When a property is marked as unique, it means that each value in that property must be unique across all instances of the model. In other words, no two instances of the model should have the same value for the specified unique property.
Model: name: "Person" properties: - name: "email" type: "String" unique: true - name: "name" type: "String"
Long Text
- It refers to a property that stores text data, such as logs or comments, associated with an instance of the model, and also to store larger text values, such as detailed descriptions, comments, or any other extensive textual information.
model: name: "Article" properties: - name: "title" type: "String" - name: "content" type: "String" longText: true
Access
- Need to ask @Nagaraju
Validation
Property validations are used to enforce rules and constraints on the values that can be assigned to a specific property. Validations help ensure that the data adheres to predefined criteria, promoting data integrity and consistency. Here's an overview of property validations:
Error Message: The "Error Message" allows you to specify a custom error message that will be displayed when the validation rule is not satisfied. You can provide a clear and descriptive error message to help users understand why the validation failed.
Expression: The "Expression" allows you to define a custom expression or condition that will be evaluated to determine if the validation rule is satisfied.
Validate On Create/Validate On Update: These control when the validation should be triggered. "Validate On Create" specifies whether the validation should occur when creating a new instance, and "Validate On Update" specifies whether the validation should occur when updating an existing instance.
Server Only: The "Server Only" indicates whether the validation should be performed only on the server side.
Model: name: "Application" properties: - name: "email" type: "String" validations: - expression: "RegExp('^[a-zA-Z][a-zA-Z0-9- ]*\$').hasMatch(it)" errorMessage: "Name is Invalid!"
Validations:
- Specifies rules and conditions to ensure data integrity and consistency.
- Ensures that the data adheres to predefined criteria, enhancing the reliability and accuracy of the application.
Key Components of Validations:
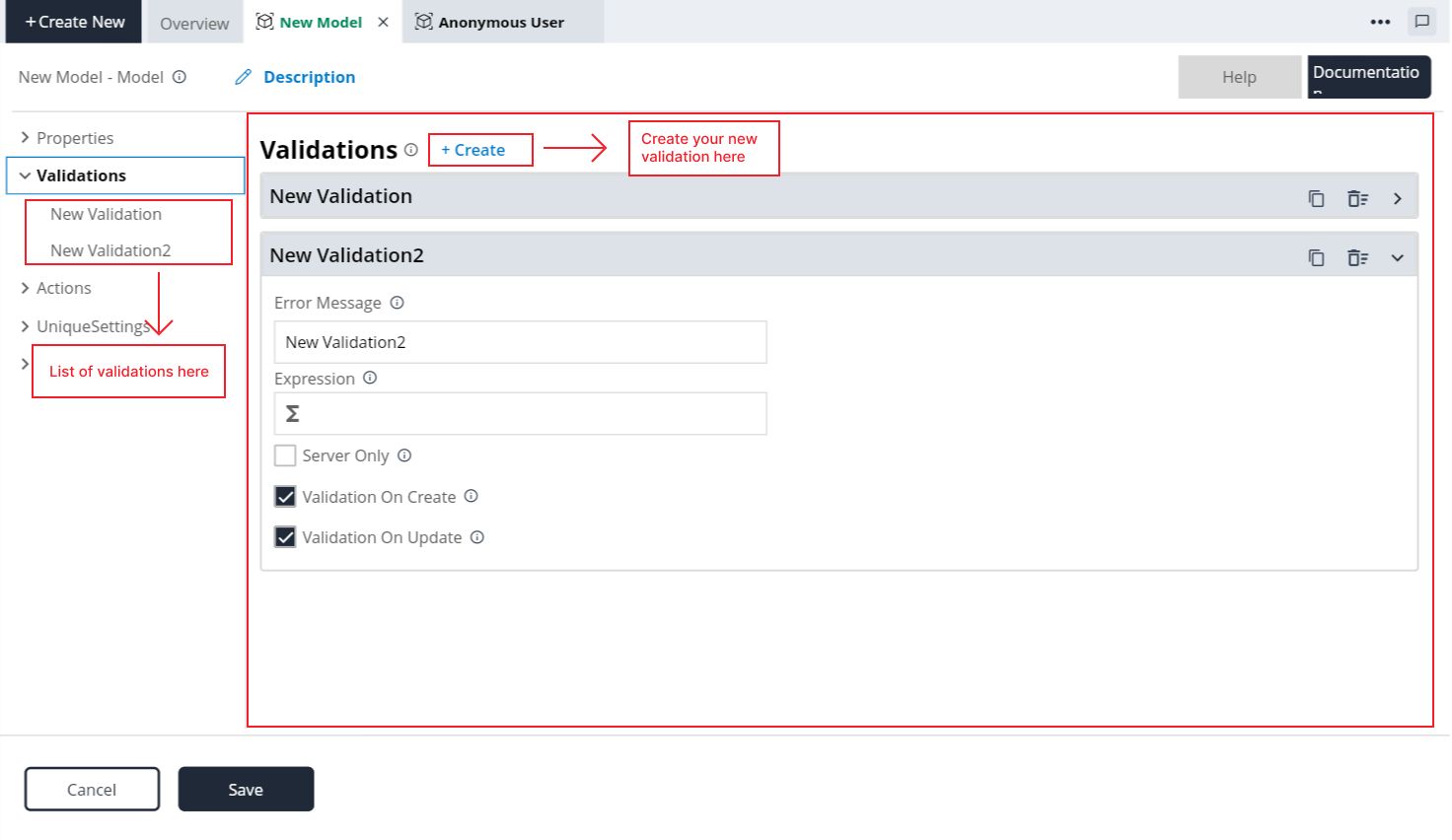
Model validations are used to enforce rules and constraints on the values that can be assigned to a specific model. Validations help ensure that the data adheres to predefined criteria, promoting data integrity and consistency. Here's an overview of model validations:
Error Message: The "Error Message" allows you to specify a custom error message that will be displayed when the validation rule is not satisfied. You can provide a clear and descriptive error message to help users understand why the validation failed.
Expression: The "Expression" allows you to define a custom expression or condition that will be evaluated to determine if the validation rule is satisfied.
Validate On Create/Validate On Update: These control when the validation should be triggered. "Validate On Create" specifies whether the validation should occur when creating a new instance, and "Validate On Update" specifies whether the validation should occur when updating an existing instance.
Server Only: The "Server Only" indicates whether the validation should be performed only on the server side.
Actions:
- Define the operations or behaviors associated with the model.
- Allows developers to specify how the data interacts with the application, including create, update, delete, user selection, schedule, create and update.
Key Components of Actions:
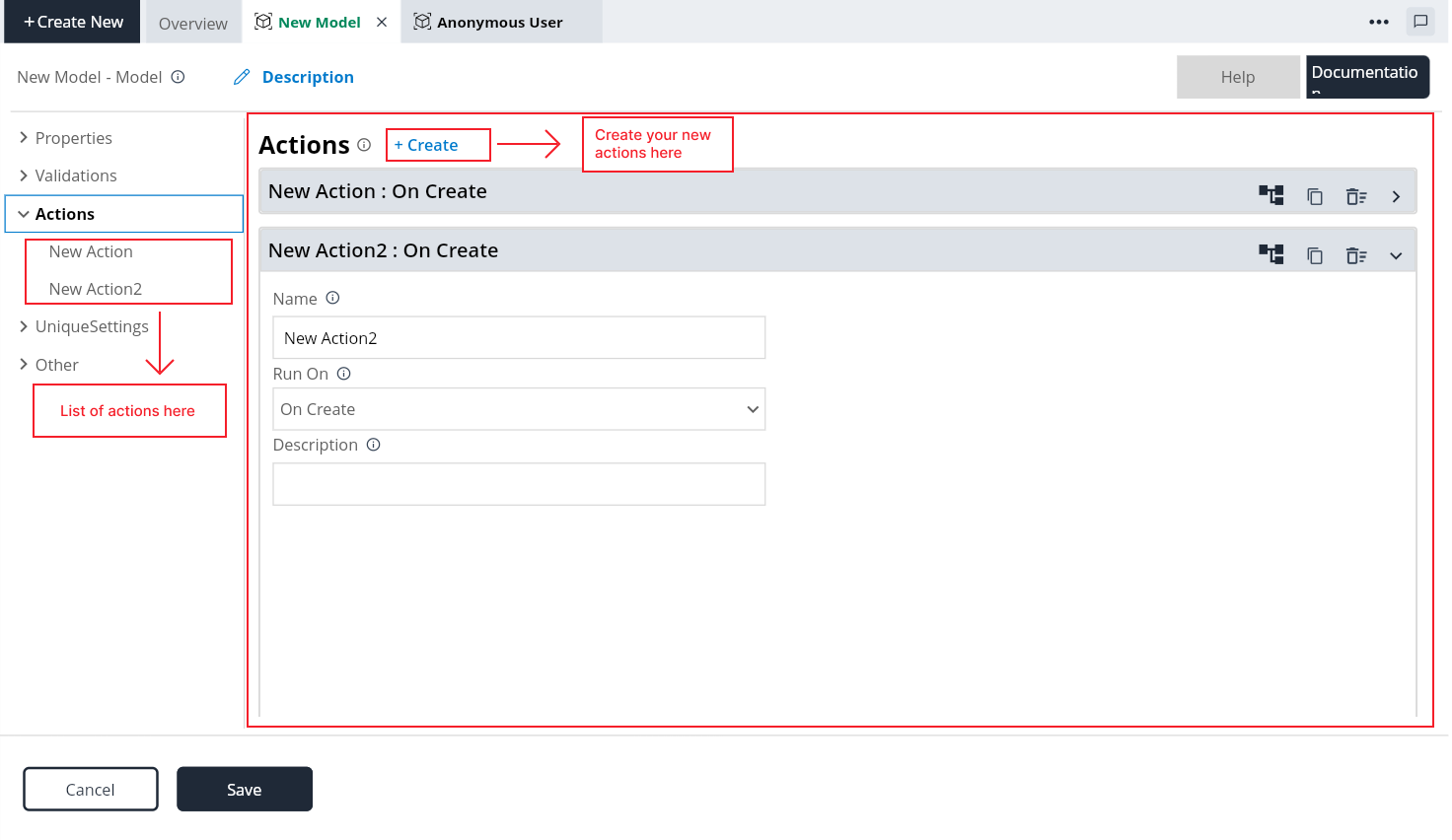
Name: The "Name" component is a unique identifier for the action. It provides a meaningful and recognizable name for the action, making it easier for developers to reference and understand the purpose of the action.
Run On: The "Run On" component specifies the context or trigger on which the action should be executed. It defines when the action should run, such as:
- On create
- On Update
- On Delete
- On User Selection
- On Scheduled
- On Create and Update
Description: The "Description" component provides a brief explanation or documentation of what the action does. It helps developers and other stakeholders understand the purpose and functionality of the action.
Code Editor: The "Code" component contains the logic and implementation details of the action. It defines the actual behavior that the action performs when triggered. Developers can write custom code in the D3E Studio's scripting language to execute specific tasks.
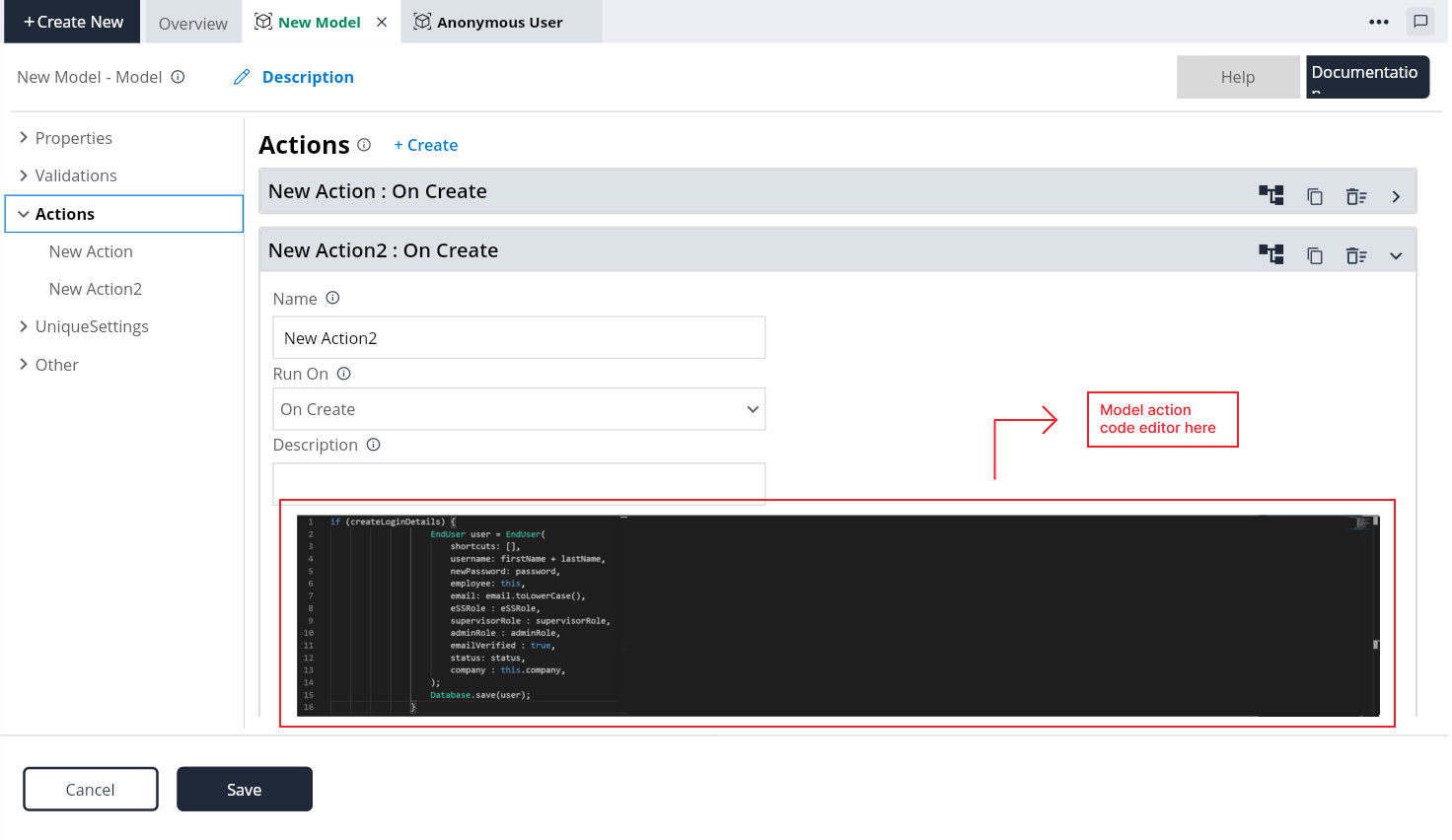
- Steps: The "Steps" component logic and implementation details of the action and Developers can write custom code in the D3E Studio's steps.
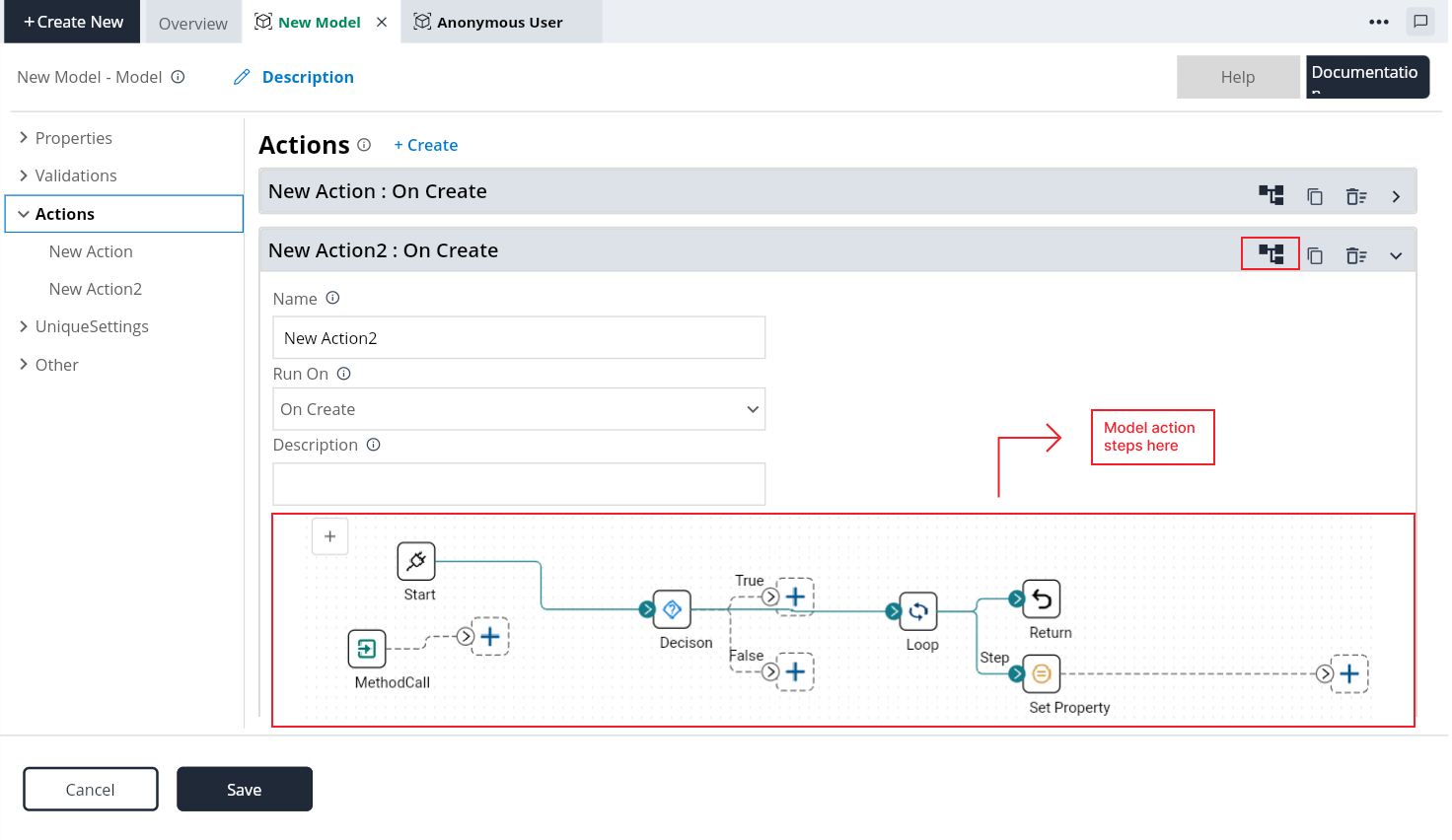
Unique Settings:
- Configurations that define the uniqueness and behavior of the model.
- This ensures that no two records in the model have the same combination of values for the selected properties.
- Additionally, you can define a custom error message to be displayed when a uniqueness
Key Components of Unique Settings:
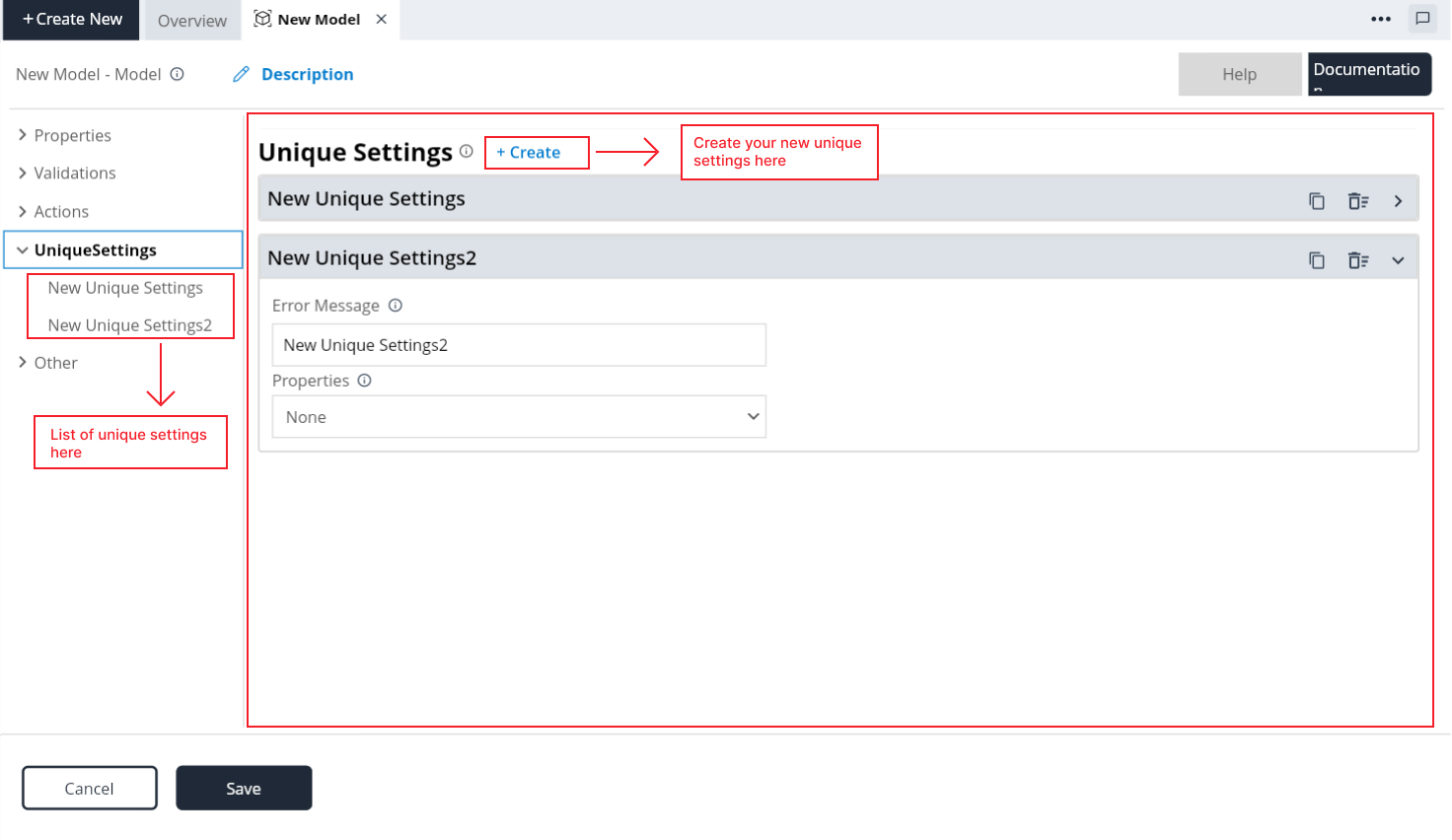
Error Message: The "Error Message" allows you to specify a custom error message that will be displayed when the validation rule is not satisfied. You can provide a clear and descriptive error message to help users understand why the validation failed.
Properties: The "Unique Settings" of a model allow you to specify a combination of properties that, when combined, must be unique in the model.
Example:
Model:
name: "Employee"
properties:
- name: "employeeId"
type: "text"
- name: "email"
type: "text"
- name: "department"
type: "text"
uniqueSettings:
- properties: ["employeeId", "email"]
errorMessage: "Combination of Employee ID and Email must be unique."
Creatable:
- Specifies whether instances of the model can be created.
Master:
- Specifies the parent model for hierarchical relationships.
- You can select a master for a model from the master dropdown.
- Master:
Parent:
- Specifies the parent model for hierarchical relationships.
- You can select a parent for a model from the parent dropdown.
Read Type:
Defines the read access type for the model.
Types of Read Type:
- Read and Write
- Write Once
- Read Only
- Local
Display:
- Determines how the model is displayed in all other objects.
Is Abstract:
- Indicates whether the model is abstract.
Is Embedded:
- Specifies if the model is embedded within another model.
Is Singleton:
- Defines whether the model is a singleton.
Is Transient:
- Indicates if the model is transient.
Need Created Date:
- Specifies whether the model requires a created date.
Need Updated Date:
- Specifies whether the model requires an updated date.
Inputs:
- Describes the various input fields associated with the model.
Key Components of Inputs:
- Name: Input is uniquely identified by a name within the model.
- Models: You have to select the model as the input.
- Required: - The "required" property inputs specifies whether a particular input must have a value assigned. If a input is marked as required, it means that when creating or updating an object based on this model, a value for this input must be provided, and leaving it empty would result in a failure, ensuring essential data is captured.
Importance of Models:
Backend Generation:
- More than 90% of the backend application is generated based on the models defined.
Data Storage:
- Models determine how data is stored in the database, representing the core structure of your application's data.
Form Relationships:
- Models are associated with forms in the application, defining the data input and output for various UI components.
Hidden Essential Models:
- Some models might not be visible in the UI but are crucial for storing essential data.
Duplicate, Edit, Delete in Models:
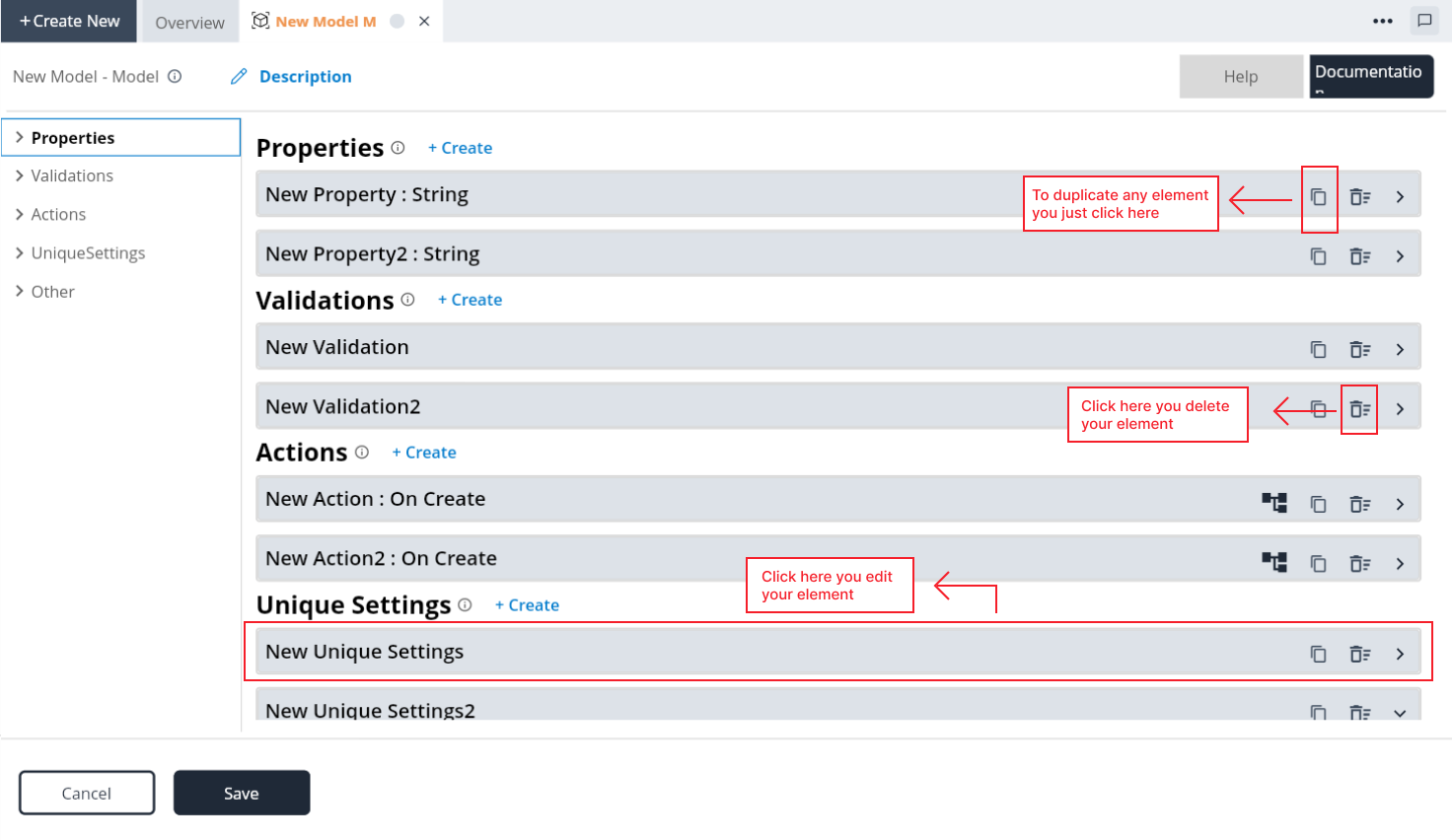
Duplicate: Properties, Validations, Actions, Unique Settings, Inputs: To duplicate any of these elements, navigate to the respective section in the model editor, locate the item you want to duplicate, and use the provided options to duplicate it.
Edit: Properties, Validations, Actions, Unique Settings, Inputs: To edit any of these elements, navigate to the respective section in the model editor, locate the item you want to edit, and tap on the item's header then it will expand to edit and update it.
Delete: Properties, Validations, Actions, Unique Settings, Inputs: To delete any of these elements, navigate to the respective section in the model definition, locate the item you want to delete, and use the provided options to remove it.
In conclusion, models in D3E Studio form the foundation for your application's data structure, influencing both the backend logic and the user interface. With properties, validations, actions, and unique settings, models provide a comprehensive framework for defining, storing, and interacting with data. Understanding the intricacies of each model component is crucial for effective application development in D3E Studio.
-
ON THIS PAGE
- Introduction
- Key Components of Model
- Importance of Models: